TableExportOptions objects hold complete descriptions of options for exporting tables to fixed-column-width or column-delimited text files. TableExportOptions objects have fields that are settable by the user to create marker lines of various styles. New TableExportOptions objects are created by calls to TableExportOptions() (e.g., myOptions = TableExportOptions()). TableExportOptions fields are described below:
TableExportOptions Field | Type | Description | Default |
---|
delimiter | string (one character) | Placed between fields if fixedWidthCol is False | '\t' (tabcharacter) |
quotedStrings | Boolean | Specifies whether to enclose text in quotes | False |
title | string | Title of the table | None |
fixedWidthCols | Boolean | Specifies whether fields are exported as fixed-width columns or fields separated by delimiter | False |
columnHeader | Boolean | Specifies whether the column headers are to be exported | True |
rowHeader | Boolean | Specifies whether the row headers are to be exported | False |
gridLines | Boolean | Specifies whether the table will be exported with text "lines" between the rows and columns | False |
The script in Example 33 creates a table from two DSS data sets and exports the table as a comma-separated-value text file.
Example 33: Filling, Displaying, and exporting a Table
from hec.heclib.dss import HecDss # for DSS
from hec.dssgui.script import Tabulate
from hec.script import TableExportOptions
file = r"C:\project\DSSVue-Example-Scripts\data\sample.dss" # specify the DSS file
dssfile = HecDss.open(file) # open the file
#read 2 records
stage = dssfile.get("/MY BASIN/RIVERSIDE/STAGE//1HOUR/OBS/")
flow = dssfile.get("/RUSSIAN/NR HOPLAND/FLOW//1HOUR//")
theTable = Tabulate.newTable() # create the table
theTable.setTitle("Test Table") # set the table title
theTable.setLocation(5000,5000) # set the location of the table off
# the screen
theTable.addData(flow) # add the data
theTable.addData(stage)
theTable.showTable() # show the table
flowCol = theTable.getColumn(flow) # adjust columns
stageCol = theTable.getColumn(stage)
flowWidth = theTable.getColumnWidth(flowCol)
stageWidth = theTable.getColumnWidth(stageCol)
theTable.setColumnPrecision(flowCol, 0)
theTable.setColumnPrecision(stageCol, 2)
theTable.setColumnWidth(flowCol, flowWidth - 10)
theTable.setColumnWidth(stageCol, stageWidth + 10)
opts = TableExportOptions()# get new export options
opts.delimiter = ","# delimit with commas
opts.title = "My Table"# set the title
fileName = "c:/temp/table.txt"# set the output file name
theTable.stayOpen()
theTable.export(fileName, opts)# export to the file
theTable.close()# close
PY
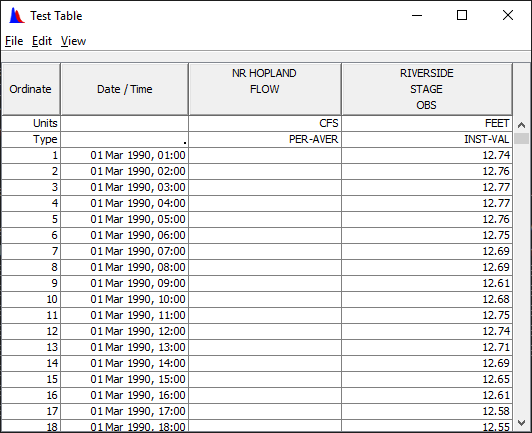